목록분류 전체보기 (351)
printf("ho_tari\n");
2024.7.29오늘 오전 이론 교육 시간에 배운 내용은 람다(lambda) 표현식의 활용이다.람다 표현식은 식 형태로 되어 있다. 함수를 간편하게 작성할 수 있어서 다른 함수의 인수로 넣을 때 주로 사용한다.lambda arguments: expressionlambda: 람다 함수를 정의하는 키워드이다.arguments: 함수의 입력 인자, 콤마로 구분하여 여러 개를 넣을 수 있다.expression: 인자를 사용하는 표현식으로, 이 표현식의 결과가 함수의 반환 값이 된다.람다 함수는 파이썬에서 간단한 함수를 한 줄의 코드로 작성할 수 있도록 해주는 익명 함수이다.람다 함수의 특징1. 이름을 지정하지 않고, 필요한 곳에 바로 작성하여 사용할 수 있다.2. 람다 함수는 작은 익명 함수이다.3. 람다 함수..
2024.7.26오늘 오전 이론 교육 시간에 배운 내용은 파이썬에서 터틀 그래픽스를 활용하는 방법이다.파이썬을 조금 해봤지만 터틀 그래픽스라는 건 처음 들어봐서 매우 신기했다.터틀 그래픽스는 그림을 그리는 모듈이고 윈도우, 리눅스, 맥 그래픽 환경에서만 동작한다.콘솔만 있는 환경에서는 사용할 수 없다.터틀 그래픽스 이후에는 PyQt에 대해서 배웠다.PyQt는 Python 프로그래밍 언어를 위한 크로스 플랫폼 GUI 툴킷이다. 이 툴킷은 Qt 라이브러리의 기능을 Python언어로 사용할 수 있도록 하는 wrapper 역할을 한다.Qt는 다양한 운영 시스템에서 동일하게 작동하는 응용 프로그램을 개발할 수 있도록 돕는 인기 있는 프레임워크이다.PyQt를 사용하면 개발자는 Qt가 제공하는 강력한 기능들을 Pyt..
2024.7.25오늘 오전 이론 교육 시간에 배운 내용은 자료구조에서 시퀀스이다.리스트, 튜플, range, 문자열의 공통점: 연속적(sequence)값이 연속적으로 이어진 자료형을 시퀀스 자료형(sequence types)라고 부름시퀀스 자료형 중에서 list, tuple, range, str을 주로 사용하며 bytes, bytearray라는 자료형도 있음시퀀스 자료형의 특징: 공통 동작과 기능을 제공시퀀스 객체: 시퀀스 자료형으로 만든 객체요소(element): 시퀀스 객체에 들어있는 각 값 오전 시간에 시퀀스 객체의 요소를 다루는 다양한 파이썬 문법을 배우고 이를 활용하여 문제를 푸는 연습을 하였다.시퀀스 객체를 잘 다루는 능력은 중요하다고 생각한다. 파이썬을 이용하여 수학이나 다양한 데이터 자료들..
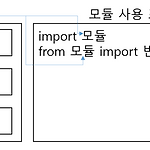
2024.7.24오늘 이론 교육 시간에 배운 내용은 모듈이다.모듈은 관련된 상수, 함수 또는 클래스 등을 모아놓은 파일이다.모듈이 필요성1. 코드 관리의 용이성 : 코드가 길어지면 한 개 파일에 모든 코드를 넣고 관리하는 것이 어려움2. 실행의 효율성 : 긴 코드를 메모리에 올려서 실행시키는 것은 비효율적3. 재사용성 : 관련된 함수나 클래스들을 한 개 파일에 묶으면 재사용이 용이해짐다른 모듈 파일을 사용하려면 import해야 함모듈_이름.함수_이름 또는 모귤_이름 형태로 사용 가능패키지(package) : 관련된 모듈을 묶어놓은 것라이브러리 : 관련된 패키지들을 모라서 한 개의 묶음으로 만든 것패키지 관리 프로그램 : 다른 사람들이 만들어둔 패키지(모듈들)을 쉽게 설치하고 사용할 수 있도록 pip라는 ..
2024.7.23오늘 이론 교육 시간에 배운 내용은 객체 지향 프로그래밍이다.오늘은 코딩적인 내용보다 이론적인 내용에 대해서 다루었다. 객체 지향 프로그래밍의 방법으로는먼저, 데이터와 코드를 객체로 함께 구성해서 한 개 자료형으로 취급하고 많은 객체 지향 언어들이 클래스(class)라는 이름으로 데이터와 코드를 묶을 수 잆는 기능을 제공한다.객체(object)는 프로그램이 실행될 때 클래스로부터 생성되는 실체이고클래스(class는 객체의 속석(변수)과 속성들을 사용하고 처리하는 기능(함수 또는 메서드)를 포함하는 코드이다. 클래스 = 속성(변수) + 기능(함수) + 인터페이스클래스는 객체를 생성하기 위한 설계도 또는 설명서이다.속성을 나타내는 변수들과 이를 사용하는 함수들로 구성된다. 또, 자료형이르모 ..
2024.7.22오늘 이론 교육 시간에 배운 내용은 딕셔너리와 집합이다.딕셔너리는 키(key)와 값(value)으로 구성된 한 쌍의 데이터를 담을 수 있는 자료구조 (map, hash라고 부르기도 함)이다.중복된 키가 포함될 수 없고 키는 수정 불가능한 것만 사용 가능하다.값은 변경이 가능하다.중괄효 {}로 표현을 한다. {키1: 값1, 키2: 값2}요소에 대한 접근은 []을 사용한다.값 추가 또는 변경이 가능하고 del()을 사용하여 삭제도 할 수 있다.집합(set) 자료구조는 수학에서의 집합을 나타내는 자료구조를 의미한다.파이썬은 집합을 표현하는 세트(set)라는 자료형을 제공한다. 세트는 합집합, 교집합, 차집합 등의 연산이 가능하다.집합은 순서가 없고 동일한 데이터가 두 개 이상 존재할 수 없다...
2024.7.19오늘 이론 교육 시간에 배운 내용은 파이썬에서의 예외 처리이다.프로그래밍을 하다보면 정말 다양한 오류들을 마주하게 된다. 이런 오류들의 종류는 다음과 같다.1. 문법 오류2. 논리 오류3. 실행 오류문법 오류는 프로그래밍 언어를 잘못 사용해서 발생한다. 파이썬이 어디서 오류가 발생했는지 알려준다.논리 오류는 문제 해결 방법을 잚못 지정한 것이다.실행 오류는 문법적/논리적으로 문제가 없는데 오류가 발생하는 것이다. 예를 들면 사용자가 정수로 변환할 수 있는 문자열을 입력해야하는데 잘못 입력했을 때, 파일을 여는데 이미 삭제되었거나 존재하지 않는 파일일 때, 파일에서 데이터를 읽거나 쓸 때, 하드디스크에 오류가 있거나 파일에 문제가 있어 동작하지 않을 수 있을 때의 오류이다.이런 오류에 대응..
2024.7.18오늘 이론 교육 시간에 배운 내용은 파이썬을 이용하여 파일을 다루는 방법이다.파일은 컴퓨터의 보조 저장 장치(ssd, hdd, odd, usb 등)에 저장되는 데이터를 담는 논리적인 저장 용기(container)이다.파일에 담을 수 있는 데이터는 종류의 제한이 없고 디지털로 표현 가능란 데이터이다. 사진, 음악, 문서 등이다.텍스트 파일은 일반적으로 사람들이 사용하는 글자들로 구성된 데이터를 담고 있다. 메모장 또는 코딩용 에디터(텍스트 편집기)를 이용해서 수정이 가능하다.바이너리 파일은 이진화된 데이터를 담고 있다. 수정하려면 전용 프로그램 또는 특화된 프로그램이 필요할 수 있다.이 두 파일의 구별 방법은 확장자를 보는 것이다. 오후 실습 교육 시간에는 파일의 입출력, 읽기, 쓰기, 문..